Renaming Columns
Renaming Columns
It’s often the case we want to rename columns in our dataframe. This is achieved very easily. Using an example of a dataset on bison mass,
# load libraries
library(lterdatasampler)
library(tidyverse)
bison <- lterdatasampler::knz_bison
head(bison)
## # A tibble: 6 × 8
## data_code rec_year rec_month rec_day animal_code animal_sex
## <chr> <dbl> <dbl> <dbl> <chr> <chr>
## 1 CBH01 1994 11 8 813 F
## 2 CBH01 1994 11 8 834 F
## 3 CBH01 1994 11 8 B-301 F
## 4 CBH01 1994 11 8 B-402 F
## 5 CBH01 1994 11 8 B-403 F
## 6 CBH01 1994 11 8 B-502 F
## # … with 2 more variables: animal_weight <dbl>, animal_yob <dbl>
To see what the existing names are, we can call the names()
function:
names(bison)
## [1] "data_code" "rec_year" "rec_month"
## [4] "rec_day" "animal_code" "animal_sex"
## [7] "animal_weight" "animal_yob"
Plains Bison
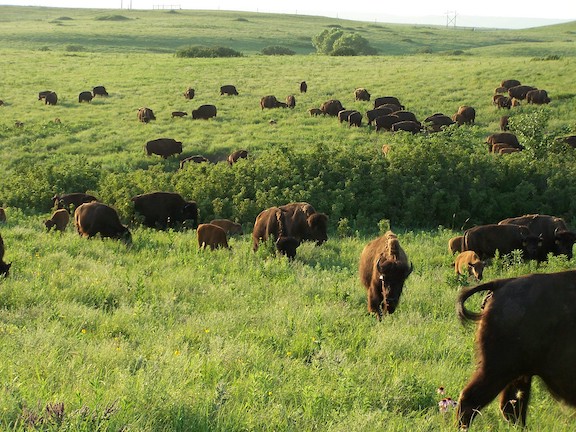
There are actually two subspecies of bison found in North America, the larger Wood bison (Bison bison athabascae), and the smaller Plains bison that we usually think of, (Bison bison bison). Males are often heavier than females, as this species engages in male-male competition for mate chioce behaviour. The data on organism size in this dataset comes from a long-term study system of a grassland ecosystem at the Konza Prairie Long-Term Ecological Research centre in northeastern Kansas.
Rename A Single Column
The Tidy method of renaming is through the rename()
function from the dplyr
package. Let’s remove the prefix “animal” from “animal_code”. In this syntax, the new name comes first, the old name second.
bison <- bison %>%
dplyr::rename(code = animal_code)
We double check with:
names(bison)
## [1] "data_code" "rec_year" "rec_month"
## [4] "rec_day" "code" "animal_sex"
## [7] "animal_weight" "animal_yob"
And we see it worked.
Rename Multiple Columns
We can rename as many columns as we want.
bison <- bison %>%
dplyr::rename(
sex = animal_sex,
weight = animal_weight,
yob = animal_yob
)
names(bison)
## [1] "data_code" "rec_year" "rec_month" "rec_day" "code"
## [6] "sex" "weight" "yob"